Toon Shader#
Color Setting#
bpy.context.scene.view_settings.view_transform = "Standard"
inner_faces_shader#
inner_faces_shader_with_hole#
Shader: inner_faces_shader_with_hole
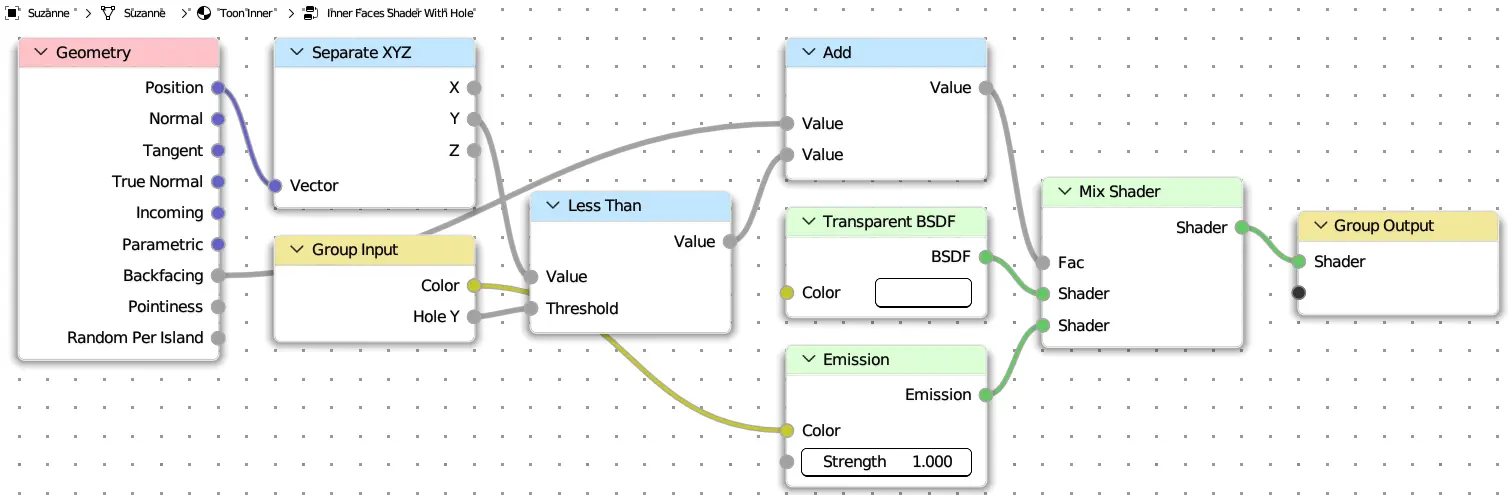
@tree
def inner_faces_shader_with_hole(color: Color, hole_y: Float = 0.5):
"""@shader"""
shader1 = BSDF.Transparent()
shader2 = BSDF.Emission(color)
fac = shader1.geometry.backfacing + shader1.geometry.position.y.less_than(hole_y)
shader = shader1.mix(shader2, fac=fac)
return shader
ramped_diffuse_color#
Shader: ramped_diffuse_color
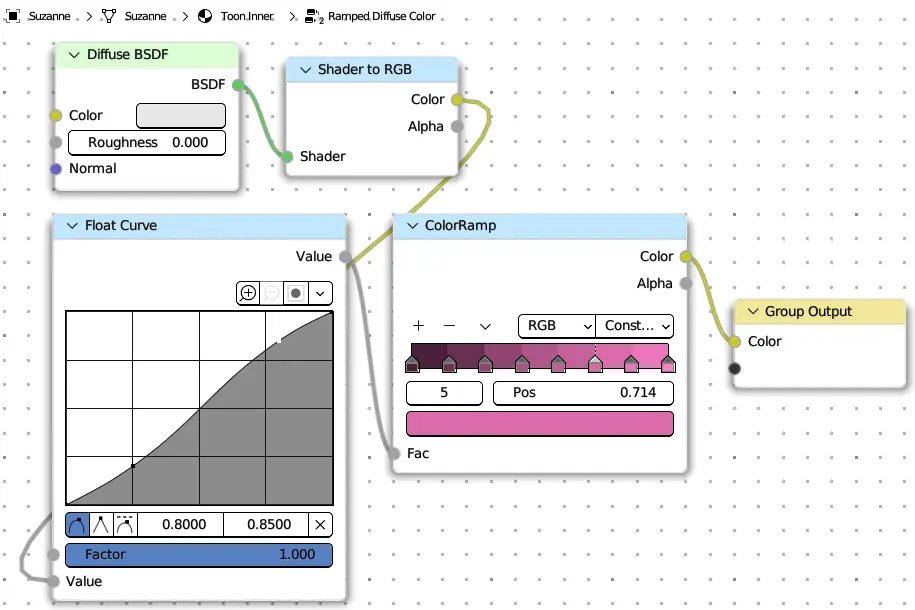
@tree
def ramped_diffuse_color():
"""@shader"""
color = BSDF.Diffuse().to_rgb().color
factor = color.Float.float_curve(points=[(0, 0), (0.25, 0.2), (0.8, 0.85), (1, 1)])
colors = []
base_color = mathutils.Color(rgb(245, 120, 193))
ramp_steps = 8
for i in range(ramp_steps):
base_color.v = (0.5 if i == 0 else i) / (ramp_steps - 1)
colors.append(base_color.copy())
# The upper for loop is equivalent to the following:
colors = rgb(76, 32, 58), rgb(106, 48, 82), rgb(146, 69, 114), rgb(175, 84, 137),\
rgb(199, 96, 156), rgb(220, 107, 173), rgb(238, 117, 188), rgb(255, 125, 201)
color = factor.color_ramp_uniform(*colors, interpolation="CONSTANT")
return color